Using The Button Form Attribute To Create Standalone Buttons In HTML
A couple of years ago, when I was first learned about Hotwire Turbo, I noticed that it was using HTML attributes to override form submissions. And, that these Turbo techniques would often associate any button with any form using these attributes. Just recently, this got me wondering if such an approach would be helpful when styling buttons that should otherwise look like anchor links.
Run this demo in my JavaScript Demos project on GitHub.
View this code in my JavaScript Demos project on GitHub.
Consider a list of links inside a <nav>
element. Imagine that the last link in this list is a log-out link. Of course, since logging out is a mutation against the system state, it should be performed as a POST
request, not a GET
request. Generally, this would mean wrapping the log-out button inside a <form>
tag.
The problem with this approach is that it gives the log-out button a different DOM (Document Object Model) structure; which makes styling the list of navigation elements more challenging. But, if we could let the log-out <button>
stand on its own, just as we do with the <a>
elements, styling would become much easier:
.nav { | |
display: flex ; | |
gap: 8px ; | |
} | |
.nav__item { | |
border: 1px solid red ; | |
border-radius: 3px ; | |
color: red ; | |
padding: 5px 10px ; | |
text-decoration: none ; | |
} |
This CSS assumes that there's a single, flat list of child elements within the nav element. No worrying about a <form>
tag that's there solely for the purposes of wrapping the <button>
.
We can do this by using the [form]
attribute on the <button>
to point to a <form>
element that is outside the <nav>
container. In the following code, notice that the <nav>
element contains a flat list of children, including the log-out button:
<!doctype html> | |
<html lang="en"> | |
<body> | |
<nav class="nav"> | |
<a href="index.htm?nav=home" class="nav__item"> | |
Home | |
</a> | |
<a href="index.htm?nav=about" class="nav__item"> | |
About | |
</a> | |
<a href="index.htm?nav=projects" class="nav__item"> | |
Projects | |
</a> | |
<!-- | |
But letting the button stand on its own, we can STYLE it in the same way that | |
we do the sibling link elements. Meaning, we don't have to worry about dealing | |
with an extra form wrapper, or use janky techniques like "display:contents". | |
--> | |
<button type="submit" form="logOutForm" class="nav__item link-button"> | |
Log-Out | |
</button> | |
</nav> | |
<!-- | |
This form has no inherent action, it's basically just a "data bag". It only gets | |
triggered when the button above is clicked. The button's [FORM] attribute makes | |
it the submit trigger for this form. | |
Note: normally, this would be a [POST] action; however, in order to make this demo | |
work on GitHub Pages, I'm using a [GET]. | |
--> | |
<form id="logOutForm" method="get" action="index.htm"> | |
<input type="hidden" name="action" value="logout" /> | |
<input type="hidden" name="submitted" value="true" /> | |
<input type="hidden" name="xsrfToken" value="abc123" /> | |
<input type="hidden" name="userID" value="10011" /> | |
</form> | |
</body> | |
</html> |
The [form=logOutForm]
attribute on the log-out button tells the browser to use the button to submit the form that's farther down in the DOM. This simplifies the structure of the <nav>
element while keeping the POST
semantics of the log-out workflow.
If we click each element in the nav, we can see that they each work. Note that for the demo, I'm actually using a GET
request on the form so that it works in GitHub Pages:
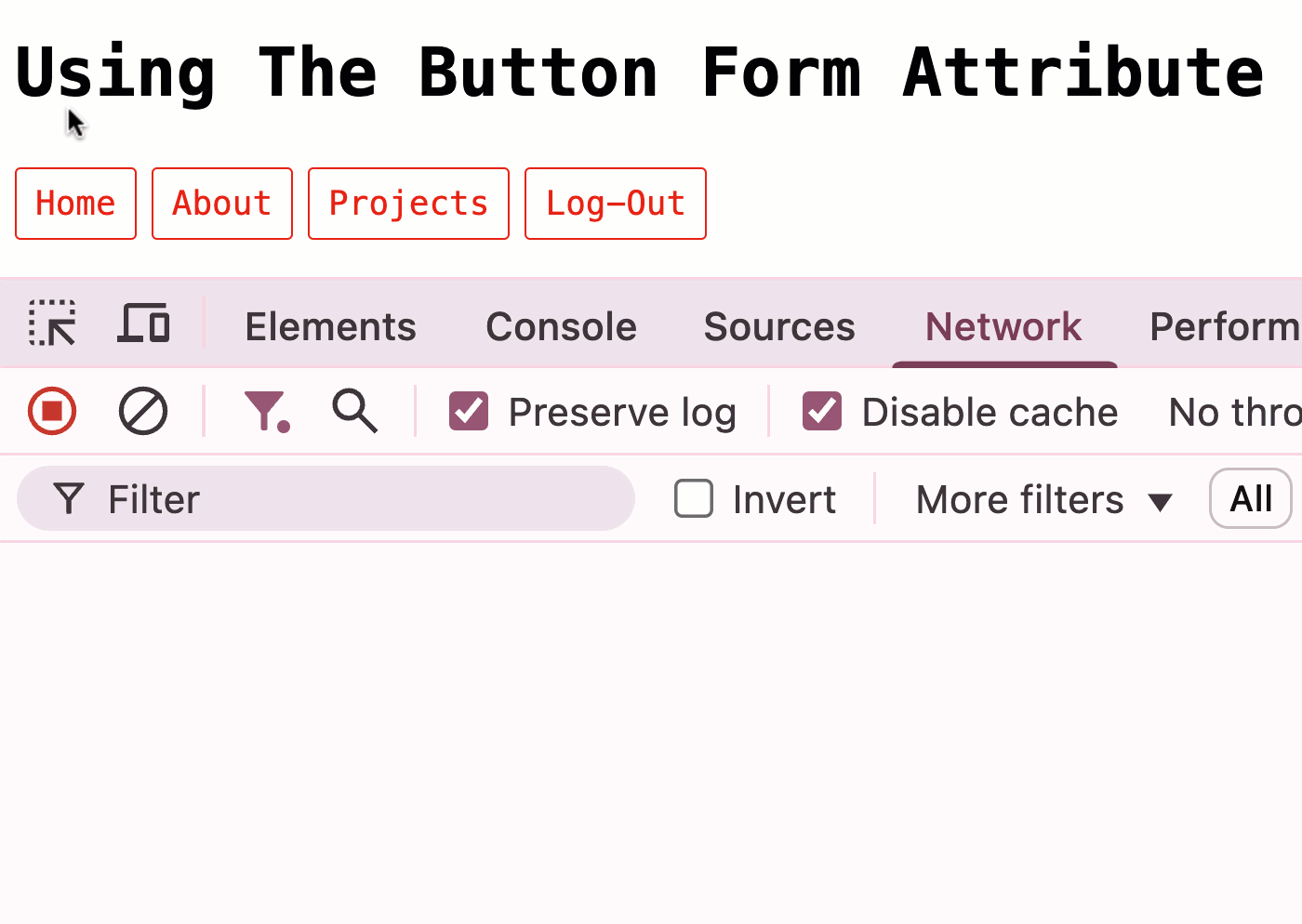
As you can see in the browser's network activity, clicking on the log-out button in the nav triggers a request to the server (again, I'm using GET
here for the demo but this should be a POST
for state mutations). By putting the button and its "parent" form in two different location, we keep all the same functionality but we make it easier to style the page.
Submit button don't only submit a form—they can also submit data along with that form by using [name]
and [value]
attributes. This got me wondering if we could use the same technique in a data grid. To explore, let's create a table in which each row has a delete button; but, each delete button will point to a single, shared form. We'll give each form submission a unique action by providing each button with a unique [value]
attribute (which references the user ID associated with the given table row):
<!doctype html> | |
<html lang="en"> | |
<body> | |
<table border="1"> | |
<thead> | |
<tr> | |
<th>ID</th> | |
<th>Name</th> | |
<th>Action</th> | |
</tr> | |
</thead> | |
<tbody> | |
<tr> | |
<td>1</td> | |
<td>Kim</td> | |
<td> | |
<button | |
type="submit" form="deleteUser" | |
name="userID" value="1" class="link-button"> | |
Delete | |
</button> | |
</td> | |
</tr> | |
<tr> | |
<td>2</td> | |
<td>Jim</td> | |
<td> | |
<button | |
type="submit" form="deleteUser" | |
name="userID" value="2" class="link-button"> | |
Delete | |
</button> | |
</td> | |
</tr> | |
<tr> | |
<td>3</td> | |
<td>Lynn</td> | |
<td> | |
<button | |
type="submit" form="deleteUser" | |
name="userID" value="3" class="link-button"> | |
Delete | |
</button> | |
</td> | |
</tr> | |
</tbody> | |
</table> | |
<!-- | |
This form has no inherent action (other than the confirm() call), it's basically | |
just a "data bag". It only gets triggered when one of the buttons above is | |
clicked. Each button's [FORM] attribute makes it the submit trigger for this form. | |
And, the [NAME/VALUE] attributes allow each button to submit a unique userID with | |
the form. | |
Note: normally, this would be a [POST] action; however, in order to make this demo | |
work on GitHub Pages, I'm using a [GET]. | |
--> | |
<form | |
id="deleteUser" | |
method="get" | |
action="index2.htm" | |
onsubmit="return confirm( 'Are you sure?' );"> | |
<input type="hidden" name="action" value="delete" /> | |
<input type="hidden" name="submitted" value="true" /> | |
<input type="hidden" name="xsrfToken" value="abc123" /> | |
</form> | |
</body> | |
</html> |
In this page, each button uses the [form]
attribute in order to trigger submission of the shared deleteUser
form element. But, each button provides a unique userID
value to be submitted along with the form. If we now run this demo and click on each button, you'll see the following output:
Note: I've removed the
confirm()
call in the GIF in order to make it simpler to run through.
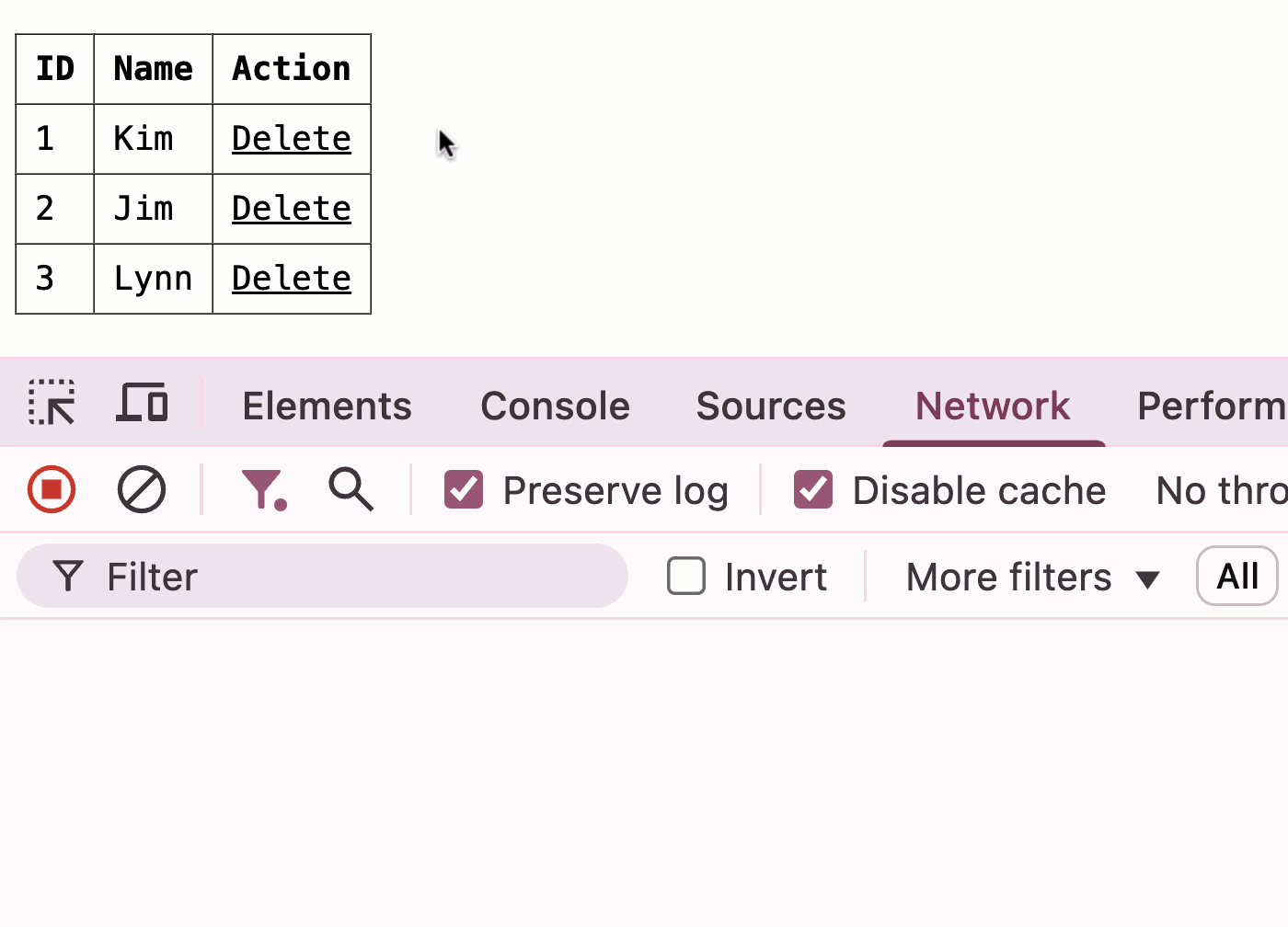
As you can see in the network activity, each of the buttons triggers submission of the same, shared form element. However, the [name]
and [value]
attributes on each button allows it to submit a unique user ID along with that form submission.
By moving the button outside of the form, we're not fundamentally changing any of the functionality of the page—we simply making parts of the page easier to style with CSS.
Considering a "ButtonTo()" Helper in ColdFusion Frameworks
Web frameworks like Ruby on Rails (RoR) and CFWheels (which is based on RoR) have helper methods, such as buttonTo()
, for generating buttons that act like links. As discussed above, the issue presented by a <button>
is that it's usually wrapped in a <form>
element. So while a buttonTo()
call might look like a linkTo()
call, if it wraps the button in a form, it can create unexpected styling challenges.
These styling challenges can be somewhat mitigated by rendering the button and the form in two different locations on the page and then associating them via the [form]
attribute:
<cfscript> | |
users = [ | |
{ id: 1, name: "Kim" }, | |
{ id: 2, name: "Jim" }, | |
{ id: 3, name: "Laura" }, | |
{ id: 4, name: "Stan" } | |
]; | |
</cfscript> | |
<cfoutput> | |
<table border="1"> | |
<thead> | |
<tr> | |
<th>ID</th> | |
<th>Name</th> | |
<th>Action</th> | |
</tr> | |
</thead> | |
<tbody> | |
<cfloop array="#users#" item="user"> | |
<tr> | |
<td> | |
#encodeForHtml( user.id )# | |
</td> | |
<td> | |
#encodeForHtml( user.name )# | |
</td> | |
<td> | |
#linkTo( | |
url = "test.cfm?action=edit&userID=#encodeForUrl( user.id )#", | |
text = "Edit" | |
)# | |
<!--- | |
Instead of outputting a FORM, this helper outputs a single BUTTON | |
element, which can be easily styled alongside any sibling elmenets | |
(such as the linkTo() anchor tag above). | |
---> | |
#buttonTo( | |
url = "test.cfm?action=delete&userID=#encodeForUrl( user.id )#", | |
text = "Delete User", | |
class = "btn btn-link" | |
)# | |
</td> | |
</tr> | |
</cfloop> | |
</tbody> | |
</table> | |
<!--- This generic form will be shared by ALL buttonTo() actions. ---> | |
<form id="buttonToForm" method="post" action="javascript:void(0)"> | |
<input type="hidden" name="xsrfToken" value="abc123" /> | |
<input type="hidden" name="submitted" value="true" /> | |
</form> | |
</cfoutput> | |
<cffunction name="buttonTo" output="true" returnType="void"> | |
<cfargument name="url" type="string" /> | |
<cfargument name="text" type="string" /> | |
<cfargument name="class" type="string" default="btn" /> | |
<!--- Point to the gneric form, but override the [action] attribute. ---> | |
<button | |
form="buttonToForm" | |
formAction="#encodeForHtmlAttribute( arguments.url )#" | |
class="#encodeForHtmlAttribute( arguments.class )#" | |
>#arguments.text#</button> | |
</cffunction> | |
<cffunction name="linkTo" output="true" returnType="void"> | |
<cfargument name="url" type="string" /> | |
<cfargument name="text" type="string" /> | |
<cfargument name="class" type="string" default="btn" /> | |
<a | |
href="#encodeForHtmlAttribute( arguments.url )#" | |
class="#encodeForHtmlAttribute( arguments.class )#" | |
>#arguments.text#</a> | |
</cffunction> |
Notice that each row in our users data-grid has both a linkTo()
and buttonTo()
call. Each of these helper functions renders a single element, which makes the UI much easier to style:
By allowing each buttonTo()
call to share the same <form>
element, we can move the form to the bottom of the page and allow only a single button to render in the original buttonTo()
location. Again, this doesn't change the semantics of the page—it only makes the rendered elements easier to style.
Want to use code from this post? Check out the license.
Reader Comments
Post A Comment — ❤️ I'd Love To Hear From You! ❤️