Associating Form Inputs With ColdFusion Validation Error Types
In my ColdFusion applications, I've never have a lot of ceremony around error handling. I simply try to catch errors as high-up in the stack as I can; and then, I use a centralized error translator to translate exceptions into a user-safe error response which I then render at the top of my form interface. It recently occurred to me that I might be able to use my user-safe error response to make my ColdFusion forms more accessible by marking form inputs as being related to certain server-side validation errors.
As much as possible, I try to keep my forms as short as possible. Which means that when I render an error message such as:
"Please enter a valid email address."
... it should be immediately clear to the user which form control is related to the given error. After all, it's probably one of only 2 or 3 inputs on the screen.
But, mapping the rendered error message onto the form input does come with some cognitive load. And, I can probably make it a little easier for the user by loosely associating one-or-more controls with the error.
To do this, I can allow each form control to provide a list of error types that might be relevant. This way, the form experience is progressively enhanced to provide more insight; but, will gracefully degrade if the server-side changes the way it does error translation.
Before we look at the client-side code, let me show you how I currently handle my ColdFusion validation. For the sake of this thought experiment, we're going to create a new User account. At the root of this workflow, I have a service component (UserService.cfc
) that represents a "User Entity". The role of this service is ensure the integrity of the data pertaining to the concept of a "user" within the system:
component
output = false
hint = "I provide service methods for the user entity."
{
/**
* I create a user with the given properties. If inputs are not valid, a validation
* error is thrown.
*/
public numeric function createUser(
required string name,
required string email,
required string password
) {
if ( ! name.len() ) {
throw( type = "App.Model.User.Name.Empty" );
}
if ( name.len() > 20 ) {
throw( type = "App.Model.User.Name.TooLong" );
}
if ( ! email.reFind( "^[^@]+@[^.]+(\.[^.]+)+$" ) ) {
throw( type = "App.Model.User.Email.Invalid" );
}
if ( email.len() > 75 ) {
throw( type = "App.Model.User.Email.TooLong" );
}
if ( password.len() < 10 ) {
throw( type = "App.Model.User.Password.TooShort" );
}
// Todo: create user entity, not the point of this demo.
return 1;
}
}
For this demo, this ColdFusion component doesn't do anything other than throw errors when we invoke the createUser()
method with invalid data. Notice that each error type
is unique. This is the error type that we're going to return to the user interface:
App.Model.User.Name.Empty
App.Model.User.Name.TooLong
App.Model.User.Email.Invalid
App.Model.User.Email.TooLong
App.Model.User.Password.TooShort
When one of these errors is thrown, we don't want to reveal the raw error information to the end-user. Instead, we want to translate this application-error into a user-safe error. For that, I use a centrally located translation service (ErrorTranslator.cfc
). This ColdFusion component performs a switch
on the error.type
and returns a payload that represents a user-safe error response:
component
output = false
hint = "I help translate application errors into appropriate response codes and user-facing messages."
{
/**
* I return a normalized, user-safe error response for the given server-side error.
*/
public struct function getResponse( required any error ) {
switch ( error.type ) {
case "App.Model.User.Conflict":
return as422({
type: error.type,
message: "That email address is already in use. Please use a different email address."
});
break;
case "App.Model.User.Email.Invalid":
return as422({
type: error.type,
message: "Please enter a valid email address."
});
break;
case "App.Model.User.Email.TooLong":
return as422({
type: error.type,
message: "Your email address is too long. It must be less than 75 characters."
});
break;
case "App.Model.User.Name.Empty":
return as422({
type: error.type,
message: "Please provide your full name."
});
break;
case "App.Model.User.Name.TooLong":
return as422({
type: error.type,
message: "Your name is too long. It must be less than 20 characters."
});
break;
case "App.Model.User.Password.TooShort":
return as422({
type: error.type,
message: "For security purposes, your password must be longer than 10 characters."
});
break;
default:
// Demo should never get here.
writeDump( error );
abort;
break;
}
}
// ---
// PRIVATE METHODS.
// ---
/**
* I generate a 422 response with the given overrides.
*/
private struct function as422( required struct overrides ) {
var response = {
statusCode: 422,
statusText: "Unprocessable Entity",
type: "App.UnprocessableEntity",
title: "Unprocessable Entity",
message: "Your request cannot be processed in its current state. Please validate the information in your request and try submitting it again."
};
return response.append( overrides );
}
}
In this deeply abbreviated example, I'm passing each error.type
value through to the user-safe error response. It's up to the application to determine which type
values are "safe" for the user to know about. If an error type should be kept private, the type
property can be overridden during this translation operation.
Now that you understand how I'm both validating data and translating errors on the ColdFusion side, let's look at a very simple account creation form. In the following ColdFusion page, the user must provide three data-points:
- Name
- Password
Some of these fields may result in multiple, unique error type
values. I'm going to use an HTML data-
attribute to define the list of error prefixes that might be relevant to the given form control. For example, on the password <input>
, I'll include:
data-error-types="App.Model.User.Password."
Then, when the ColdFusion page renders, I'll use some client-side JavaScript to query the rendered DOM (Document Object Model) and look for inputs that reference the current error type
. In this demo, I'm going to add an is-error
CSS class that will help to highlight the field for better insight.
This simple form performs a POST
back to itself. The form submission is processed at the top of the CFML template and the resultant errorResponse
is used to render both an error message above the form and the aforementioned JavaScript below the form.
<cfscript>
param name="form.name" type="string" default="";
param name="form.email" type="string" default="";
param name="form.password" type="string" default="";
param name="form.submitted" type="boolean" default=false;
userService = new UserService();
errorTranslator = new ErrorTranslator();
errorResponse = "";
if ( form.submitted ) {
try {
// Not all validation can be done at the "entity service" layer. Some
// validation must be performed across entities and must be performed at a
// higher level in the application. For the demo, we'll just hard-code a
// uniqueness check for email.
if ( form.email == "ben@test.com" ) {
throw( type = "App.Model.User.Conflict" );
}
newID = userService.createUser(
name = form.name.trim(),
email = form.email.lcase().trim(),
password = form.password.trim()
);
// Yay, user created successfully (not the point of this exploration).
} catch ( any error ) {
errorResponse = errorTranslator.getResponse( error );
}
}
</cfscript>
<cfoutput>
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<link rel="stylesheet" type="text/css" href="./main.css">
<style type="text/css">
.is-error {
box-shadow: inset 0px 0px 0px 4px hotpink ;
}
</style>
</head>
<body>
<h1>
Sign-up Form
</h1>
<!--- If there is a server-side error response, render the user-safe message. --->
<cfif isStruct( errorResponse )>
<p role="alert" aria-live="assertive">
#encodeForHtml( errorResponse.message )#
</p>
</cfif>
<form method="post" action="./test.cfm">
<input type="hidden" name="submitted" value="true" />
<p>
<label for="target--name">
Name:
</label>
<input
id="target--name"
type="text"
name="name"
value="#encodeForHtmlAttribute( form.name )#" data-1p-ignore
data-error-types="
App.Model.User.Name.Empty
App.Model.User.Name.TooLong
"
/>
</p>
<p>
<label for="target--email">
Email:
</label>
<input
id="target--email"
type="text"
name="email"
value="#encodeForHtmlAttribute( form.email )#" data-1p-ignore
data-error-types="
App.Model.User.Email.
App.Model.User.Conflict
"
/>
</p>
<p>
<label for="target--password">
Password:
</label>
<input
id="target--password"
type="password"
name="password" data-1p-ignore
data-error-types="App.Model.User.Password."
/>
</p>
<p>
<button type="submit">
Create Account
</button>
</p>
</form>
<!---
If there's a server-side processing error, we need to serialize it into the
client-side code such that we can query the DOM and look for inputs that
appear to match the server-side validation error.
--->
<cfif isStruct( errorResponse )>
<script type="text/javascript">
var errorResponse = JSON.parse( "#encodeForJavaScript( serializeJson( errorResponse ) )#" );
var formControls = document.querySelectorAll( "[data-error-types]" );
for ( var control of formControls ) {
// If any of the embedded prefixes appear in the server-side error
// type, mark this form control as being error-related.
for ( var prefix of extractPrefixes( control.dataset.errorTypes ) ) {
if ( errorResponse.type.startsWith( prefix ) ) {
control.classList.add( "is-error" );
break;
}
}
}
/**
* I break the [data-error-types] attribute into an array of types.
*/
function extractPrefixes( dataAttribute ) {
return dataAttribute.split( /[,\s]+/g )
.filter( match => match )
;
}
</script>
</cfif>
</body>
</html>
</cfoutput>
As you can see in the bottom of the page, I'm serializing the errorResponse
into the JavaScript context. Then, I'm matching the errorResponse.type
value against all of the prefixes defined within the data-
attributes. And, if any match, I append the is-error
CSS class.
Now, if I run this ColdFusion page and submit the form with progressively more information, I see the following experience:
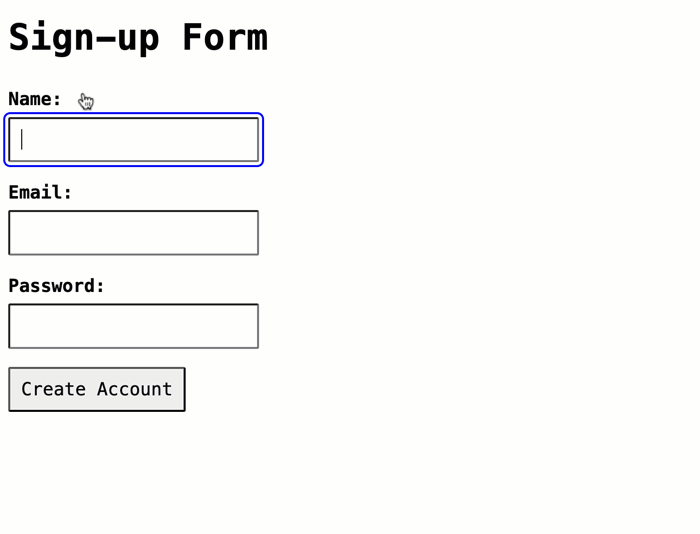
Notice that in addition to the error message that is rendered at the top, the is-error
CSS class adds an inset shadow to the relevant, problematic form field.
Using a shadow to show validation errors is problematic for people with color-blindness; so, I'd likely need to find a different (or additional) way to signal the issue. But this exploration was less about the rendering of the error and more about the association of the form controls to the ColdFusion error response.
I'm liking this technique because:
It's completely optional. As long as I always render the user-safe error response at the top, the form is usable and the errors should be relatively easy to debug. As I mentioned earlier, I keep my forms rather small; so, any objection that entails, "What if I have a form with 200 inputs on it" is immediately irrelevant for 99.999% of all websites.
It degrades gracefully. If the error
type
values change, I may lose some of the field-specific targeting; but, the error response is always rendered at the top. As such, the form remains usable.It's very simple. I don't have to deal with configuration files or build steps that introspect the database or any nonsense like that.
Right now, I'm dynamically injecting a CSS class. But, this might not be sufficient in the long run. I could always move from a client-side rendering to a server-side rendering using something like a ColdFusion module / custom tag. Imagine having an explicit tag next to each input:
<p>
<label for="target--email">
Email:
</label>
<input
id="target--email"
type="text"
name="email"
value="#encodeForHtmlAttribute( form.email )#" data-1p-ignore
/>
<!--- Will only render if the prefixes match. --->
<cfmodule
template="/tags/field-error.cfm"
errorResponse="#errorResponse#"
prefixes="#[
'App.Model.User.Email.',
'App.Model.User.Conflict'
]#"
/>
</p>
Clearly this is a lot more work than a generically applied CSS class. But, it would allow for a much more robust implementation.
And, I'm sure there are myriad of other approaches (such as using an HTML <template>
tag instead of a CFModule tag). The goal here isn't to find the perfect solution (spoiler alert, one doesn't exist); the goal here was really to see if I could find a solution that loosely ties a form control to an error type
returned by the ColdFusion server. And that seems quire reasonable to me.
Want to use code from this post? Check out the license.
Reader Comments
In my exploration, I'm adding an
is-error
CSS class to the input field in question. But, I was just reading this article over on Smashing Mag about accessible form field validation by Sandrina Pereira, and she discusses putting thearia-invalid="true"
attribute on the input. This way, the screen reader / assistive technology will announce the field as invalid when the user re-focuses the input. I could certainly update my approach to use[aria-invalid="true"]
as a CSS selector to apply the visual changes.That said, in her article, she also mentions that a non-color-based validation approach is also a must (I allude to that with my comment about color-blindness and she validates my assumption).
I (mostly) like it. I don't love that the server error type has to exactly match the
data-error-types
because that tightly couples the two and requires some back/forth to ensure they're married up properly. But, it's straight forward and simple and most importantly doesn't blow up if you map it incorrectly, which is great.I might also do the user a solid by adding an "autofocus" to the first error element.
I believe programmers tend to dislike form validation in general, and I get it. But I find it fascinating how something so seemingly simple at first glance can become so complex in practice. And there are so many ways to do it...which is probably why programmers tend to dislike the topic :)
@Chris,
I totally get the dislike of the tight coupling between the server and the client. Trust me, I don't like it either 😆 But, honestly, I have no idea how else you get there. No matter what, I think you're gonna end up with tight coupling in whichever approach you use because, ultimately, the source-of-truth is the back-end; and, we're just trying to figure out how to present information on the front-end.
This is why I feel so strongly about doing so little error handling on the client-side and deferring all of it to the server-side. Then, at least, you can always count on the error message at the top being correct (since the source-of-truth is the server-side handling of logic). The more logic you build into the client-side workflow, the more you'll have to keep logic up-to-date in two different places.
Honestly, if there's a better / easier way to do this, I'm all for it. It's not an area of app development that I spend a lot of time thinking about.
@Ben Nadel,
Yeah, I get it and agree there will always be some coupling that needs to happen. My thoughts are around minimizing it in a natural way. You already need to name the input. That name closely and naturally aligns with the
errorResponse.type
which means there's probably an opportunity to eliminate thedata-error-types
attribute entirely.That's just my first thought.
The other thing that I do a lot of hand wringing about is how much of the information processing I want do on the server-side and how much I want to do on the client-side. For example, in my Incident Commander app, I do the "top of form" error message display in ColdFusion on the server-side. But, then I've ended up doing some DOM-manipulation on the client-side.
The way it works is that my error message at the top is a custom tag that has a
<template>
internally:Once this renders and Alpine.js wires-up the controllers, then it grabs that
$refs.fieldMessage
reference, clones it, and prepends it to the relevant fields based on the[data-error-types]
attribute matching outlined in the blog post.I don't love spreading the logic across several places; but, it also makes writing the Form easier since I don't have to explicitly include error rendering logic in each form field. It's messy. But, it's also has few moving parts.
Uggggg, there are no solutions! Only trade-offs on top of trade-offs on top of trade-offs 😆
@Ben Nadel,
Totally! It's whatever ergonomic makes the most sense to you. But I find that the less indirection there is, the kinder I've been to future me. With error messages, if I've forgotten where that's set...I'll do a search for the specific error message. Hopefully it'll contain something searchable not just "Fail". Haha. I might also inspect the page for context etc. The easier it is for me to connect B (the result) to A (the source) the better IMHO.
In your case, it looks like you're co-locating the template near the message output, so that's not too difficult to find. But the template could be anywhere which makes it more difficult to connect the dots.
Trade-offs! It's a love/hate relationship 🙃
I always liked ValidateThis ... I like where you are headed. I'm curious about the pros/cons of showing each error individually (and having to fix each one) vs highlighting all the fields at once?
@Jim,
Validating one field at a time is a greatly simplified workflow (at least to me). You don't have to have any additional concept of a collection of errors. And, the server side code gets to handle the "happy path" proceduraly and then rely on error throwing to handle the "sad path."
If we assert, for a moment, that the validation has to be performed inside the "application core" and not in the "controller layer" since there will always be validations that are business requirements, and not just data wrangling requirements, then it means that there's going to be a Function that handles the form processing intent. Example:
core.createNewUser(...)
.For this application core method to report errors for several fields at one time, it would have to return some higher-level abstraction like a "Create New User Result" object that either has success data (ex, the new user ID) or the error data (ex, the collection of all fields that were invalid and/or business rules that were violated such as email address uniqueness).
Which, in turn, would mean that the field validation results would have to be aggregated into some collection internally. And then you'd also have to decide how deep to go on the validation before short-circuiting the flow. For example, if the email address isn't valid, there's not point in also checking for uniqueness of email.
To try and compose all of this data into a singular result-set adds a lot of conditional logic and and lot of control flow. And, all of this is greatly simplified if you just assume the happy path and then
throw()
at the first indication of a problem.The thing that I always try to keep a perspective on is that most forms should be simple and force the user into the "pit of success". Meaning, it should be hard for a user to do the wrong thing. As such, most forms should be processed without error; and having to report an error should be the outlier case.
When people push back and they're like, "I have a form that has a 100 fields on it and having to report back 100 errors, one at a time, is going to be terrible." ... to which I'm like, yeah, a form with 100 fields is terrible no matter how you do the error handling. The problem there isn't the error handling, it's the workflow itself.
With all that said, I'll take a look at the
ValidateThis
library 😀 I vaguely remember that from years ago - I think it was from Bob Silverberg if my memory serves me.@Ben Nadel,
I've been down the path of reporting all validation errors at once. Its my preferred UX model, but the DX does get tricky and complex quickly. I believe in giving the user all the information they need in order to be successful for the next submit. Otherwise they can be in a frustrating "ugh, now what?" loop when the form is (repeatedly) returned to them with yet another thing to fix.
I also believe that we should never require the input to be in a certain format to be valid. For example, if the user enters their phone number as...
All should be valid. We should never force the user to enter to a particular way because we can recondition the data on the server whichever way we like.
I love the pointer to the ValidateThis library. When I looked it up, I realized it's a Team CF Advance initiative/repo. I don't remember how I became part of their coldfusion team, but I see that I'm a member every time I log into GitHub. I don't think I've ever participated, it doesn't look like there's been any activity since 2017. I have to check that library out though, looks cool!
I don't think I've heard of the CF Advance team. Looks like they have a good number of repositories / projects. It's a shame it didn't get more attention.
Also, +1000 on making form inputs as flexible as possible.
Post A Comment — ❤️ I'd Love To Hear From You! ❤️
Post a Comment →