Using Multiple Break Statements In A Single Case Block In JavaScript
This morning, I was trying to learn how to use Redux when I found myself in a situation in which I wanted to break out of a reducer Case block early based on a dynamic condition (whether or not an item with the given ID could be found). If I was in a vanilla function, I'd simply create a "guard condition" with a return statement inside of it; but, I didn't want to return out of the reducer [function] - just the case. Then it occurred to me, maybe a Case block could employ multiple break statements in the same way that a function can employ multiple return statements.
Run this demo in my JavaScript Demos project on GitHub.
To test this, I created a function that would test a given expression against two sets of Case block. Each case block would match against three values in a range; and, it would console-log each value, in the range, up to the given value; but, it will "break" out of the case when it reaches the given value.
It's probably easier to just look at the code:
<!doctype html>
<html>
<head>
<meta charset="utf-8" />
<title>
Using Multiple Break Statements In A Single Case Block In JavaScript
</title>
</head>
<body>
<h1>
Using Multiple Break Statements In A Single Case Block In JavaScript
</h1>
<script type="text/javascript">
// Test values against the various ranges.
[ "A", "B", "C", "D", "E", "F", "G" ]
.forEach( testExpression )
;
// I test the given expression across different groups of values to see how
// multiple Break statements behave within a single Case block. This is akin
// to using multiple Return statements in a Function (which I happen to think
// is a wonderful feature of the language).
function testExpression( expression ) {
switch ( expression ) {
case "A":
case "B":
case "C":
console.log( "Testing " + expression + " in [ A - C ]:" );
console.log( "A" );
// Try to break out of case early.
if ( expression === "A" ) {
break;
}
console.log( "B" );
// Try to break out of case early.
if ( expression === "B" ) {
break;
}
console.log( "C" );
break;
case "D":
case "E":
case "F":
console.log( "Testing " + expression + " in [ D - F ]:" );
console.log( "D" );
// Try to break out of case early.
if ( expression === "D" ) {
break;
}
console.log( "E" );
// Try to break out of case early.
if ( expression === "E" ) {
break;
}
console.log( "F" );
break;
}
}
</script>
</body>
</html>
As you can see, within each case "range", I'm only logging-out up to the given letter; then, I'm attempting break out of the case with a conditional break statement. And, when we run the above code, we get the following output:
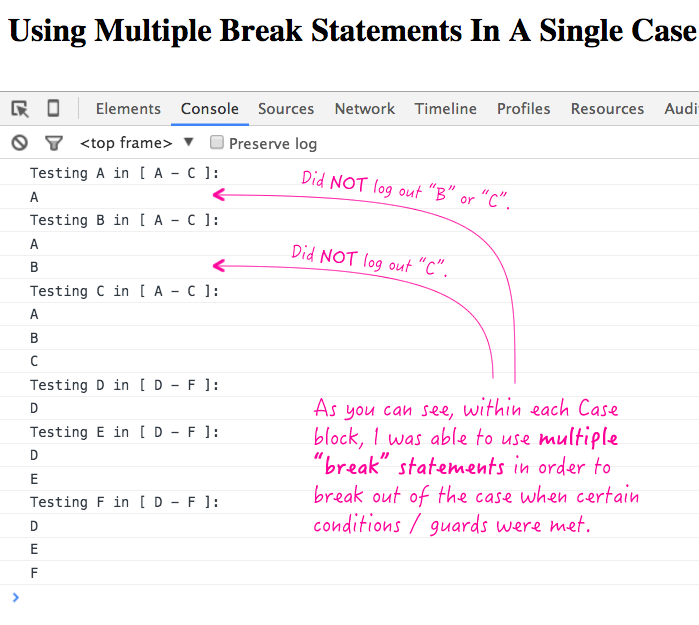
Very cool! The conditional break statement, inside of the if-blocks, worked just as we had hoped. You can use multiple break statements inside a single case block in JavaScript and it will act just like multiple return statements inside of a single function. I'm sure there are people who will say that this is a horrible idea, just as they would likely say this about multiple return statements. But, for guard logic, I think this makes for a wonderful feature of the language.
Want to use code from this post? Check out the license.
Reader Comments
great informative article...
Thank you..
@Manohar,
Glad you enjoyed :)
This reminds me of [Duff's Device](https://en.wikipedia.org/wiki/Duff's_device).
A small note regarding the term "case block": According to the spec (www.bennadel.com/blog/2986-using-multiple-break-statements-in-a-single-case-block-in-javascript.htm), the switch statement contains a *single* case block, which in turn contains one or more case clauses. The case clause finally contains a list of statements (which is optional). So, it would be more correct to say "case clause" instead of "case block" in your article.
@Šime,
The correct URL: http://tc39.github.io/ecma262/#sec-switch-statement
I much needed this bit of knowledge - thanks again to you Ben.