Using The Elvis / Null Coalescing Operator To Loop Past Array Boundaries In Lucee CFML 5.3.7.47
The Elvis / null coalescing operator (?:
) in Lucee CFML allows us to evaluate expressions that have fallback values if the left operand results in a null / undefined value. It's a powerful operator; and, can even replace the safe-navigation operator under certain circumstances. One place that I've been using it lately is when looping over an Array wherein I need to "cycle back" to the opposite end of the array upon hitting a boundary condition. I feel like it expresses the intent of the code quite cleanly. As such, I wanted to make a quick demo in Lucee CFML 5.3.7.47.
Imagine creating a linked-list or a tree structure in which each node in the structure has a reference to both the Next and Previous nodes in the same structure. In some cases, the "natural" next and previous nodes will be null; and, in those cases, we can "wrap around" to the other side of that structure by using the null coalescing operator.
Here's a very simple version of this approach:
<cfscript>
letters = [ "A", "B", "C", "D", "E" ];
linkedLetters = letters.map(
( letter, i ) => {
return([
current: letter,
// We can use the null-coalescing operator to safely wrap around the
// bounds of an array. When the incremented / decremented index results
// in an undefined array item, it means that we've hit a boundary
// condition and we need to fallback to the item at the opposite end of
// the array (either the first or last item depending on the direction).
nextLetter: ( letters[ i + 1 ] ?: letters.first() ),
prevLetter: ( letters[ i - 1 ] ?: letters.last() )
]);
}
);
dump( linkedLetters );
</cfscript>
As you can see, we're calculating the nextLetter
and prevLetter
values by adjusting the index (i
); and, if that goes out-of-bounds, we simply "cycle back" to the other side of the collection by using either .first()
or .last()
, respectively. And, when we run this ColdFusion code, we get the following output:
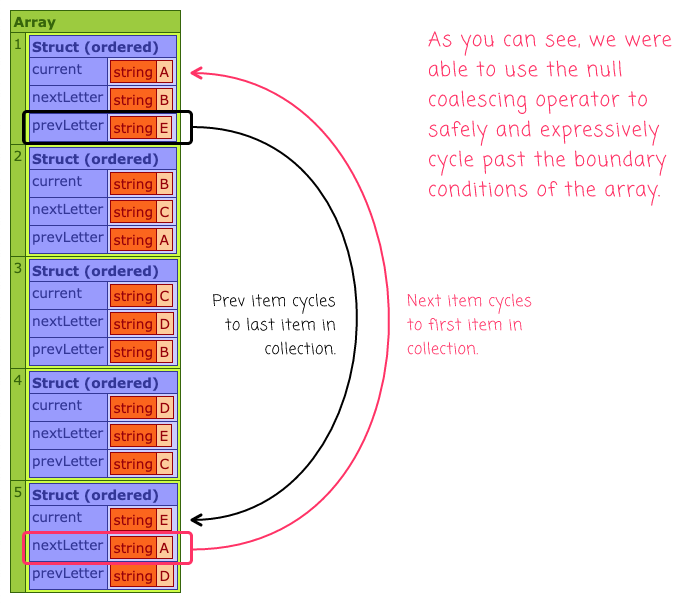
As you can see, the previous letter for A
is E
; and, the next letter for E
is A
. At each extrema of the array, we were able to use the Elvis / null coalescing operator to safely and expressively cycle back to the opposite extrema.
The null coalescing and safe-navigation operators have become such a critical part of my ColdFusion methodology. The expressiveness of these operators has really helped me to simplify a lot of my own Lucee CFML code.
Want to use code from this post? Check out the license.
Reader Comments