Binding Multiple ngSwitchWhen Cases To The Same Value In AngularJS
Earlier this morning, when I was looking into the priority changes for ngSwitchWhen in AngularJS 1.3, I noticed something odd - the ngSwitchWhen directive was "pushing" its transclusion function onto an array. In AngualrJS 1.0.8, this operation was a straight assignment. It seems that as of AngularJS 1.2, you can now have multiple ngSwitchWhen cases bound to the same value in the context of a single ngSwitch directive.
Run this demo in my JavaScript Demos project on GitHub.
I can't really think of a use-case for this feature off the top of my head. But, since it exists, I figured I'd give it a quick try in case something occurs to me later. In the following demo, I have an ngSwitch directive that contains 6 ngSwitchWhen bindings. Three of these cases are bound to "true" and 3 of them are bound to "false."
<!doctype html>
<html ng-app="Demo">
<head>
<meta charset="utf-8" />
<title>
Binding Multiple ngSwitchWhen Cases To The Same Value In AngularJS
</title>
<link rel="stylesheet" type="text/css" href="./demo.css"></link>
</head>
<body ng-controller="AppController">
<h1>
Binding Multiple ngSwitchWhen Cases To The Same Value In AngularJS
</h1>
<!--
Notice that we have multiple ngSwitchWhen "case" statements that all bind to
the same value in the ngSwitch directive.
-->
<ul ng-switch="showingEven">
<li ng-switch-when="false">
1 <em>is odd</em>
</li>
<li ng-switch-when="true">
2 <em>is even</em>
</li>
<li ng-switch-when="false">
3 <em>is odd</em>
</li>
<li ng-switch-when="true">
4 <em>is even</em>
</li>
<li ng-switch-when="false">
5 <em>is odd</em>
</li>
<li ng-switch-when="true">
6 <em>is even</em>
</li>
</ul>
<p>
<a ng-click="toggleEvenOdd()">Toggle Even / Odd</a>
</p>
<!-- Load scripts. -->
<script type="text/javascript" src="../../vendor/angularjs/angular-1.3.8.min.js"></script>
<script type="text/javascript">
// Create an application module for our demo.
var app = angular.module( "Demo", [] );
// -------------------------------------------------- //
// -------------------------------------------------- //
// I control the root of the application.
app.controller(
"AppController",
function( $scope ) {
// I determine if we're showing the even elements (or the odd by omission).
$scope.showingEven = false;
// ---
// PUBLIC METHODS.
// ---
// I toggle between showing odd and show even items.
$scope.toggleEvenOdd = function() {
$scope.showingEven = ! $scope.showingEven;
};
}
);
</script>
</body>
</html>
When the page first loads, we are set to show the odd values. And, as such, all three ngSwitchWhen cases that were bound to "false" are visible:
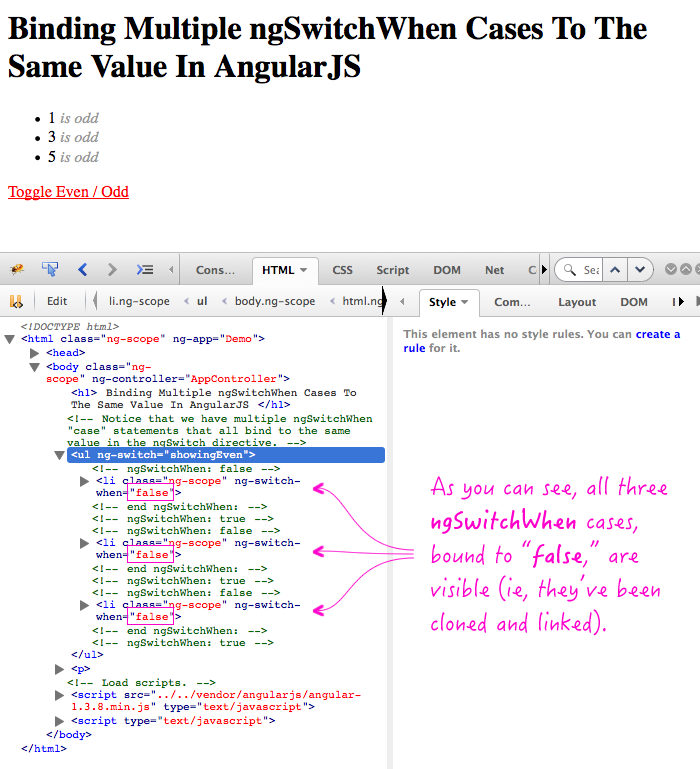
As you can see, all three ngSwitchWhen cases, bound to the same value, were all visible. This means that the ngSwitch directive cloned and linked each instance. Like I said, I am not sure what a good use-case for this is - if anyone is using this in production, I'd love to know what they're using it for.
Want to use code from this post? Check out the license.
Reader Comments